Python manage.py createsuperuser choose a username and create a password. To register a model in the Django site admin, go to the admin.py inside your app and write: admin.site.register. Don't forget to import your model: from.models import. After that, should be able to login in the django site admin and see your model. Django Tutorial in Visual Studio Code Django is a high-level Python framework designed for rapid, secure, and scalable web development. Django includes rich support for URL routing, page templates, and working with data. In this Django tutorial, you create a simple Django app with three pages that use a common base template. Django is a high-level Python framework designed for rapid, secure, and scalable web development. This tutorial explores the Django framework in the context of the project templates that Visual Studio provides to streamline the creation of Django-based web apps. In this tutorial, you learn how to.
Slack Chat:https://django-vscode.slack.com/ OverviewInstallation Mode
or, more simple
Snippets for Django templates
...and some non-official stuff:
Snippets for Django Form
Snippets for Django model fields
Snippets for Django Rest Framework
Snippets for Django Models
We work hard to add more features for this Extensions, this extension will be updated |
Visual Studio the text editor is known as Visual Studio Code is Microsoft’s free text editor that runs on Windows, Linux, and macOS. It’s a recent entrant to the market; Microsoft released the product as a public preview at the end of 2015, posting the open source code to Github, before making it available as a general release in April 2016.
Despite its newbie status, Visual Studio Code has rapidly gained popularity among developers. Some may argue that it is not a real IDE, but merely an advanced text editor. But in my opinion, after installing a number of extensions, it becomes almost a full-fledged IDE with very rich functionality.
Additionally, despite being an Electron-based application, it is quite lightweight and responsive (in contrast to for example Atom, which is very slow and resource intensive).
This tutorial will go through Installation and setup of the VS code for Python and Django projects on your machine.
Installing Visual Studio Code
Visual Studio Code is a free text editor so to download it you just have to visit their official site and download the file depending on your operating system. So visit Vscode’s-website and download the latest stable build for your OS and once the download is finished install the editor and launch the app.
The best thing about VScode is that it comes with a built-in terminal which comes handy for Django projects press Ctrl+Shift+`
to invoke the terminal.
Note that in windows powershell may seem weird for new users it is recommended to use Python debug console or CMD.
Configuring Python
To enjoy Pythonic features such as Linting, Debugging (multi-threaded, remote), Intellisense, code formatting, refactoring, unit tests, snippets, and more you need to install Python extension for Vscode.
To install an extension press Ctrl+Shift+x
or click the extension icon.
Now search for Python and install the one published by Microsoft.
Adding Extension For Django
Search for Djaneiro
this extension provides a collection of snippets for Django templates, models, views, fields & forms ported from Djaneiro for SublimeText.
Selecting Python Environment
Press CTRL+SHIFT+P(CMD+SHIFT+P for MacOS) and type Python: Select Interpreter
and select the environment for your project. You can see the active environment at the bottom left of the editor.
Installing Theme
The default Vscode theme is great in itself however there are plenty for fabulous free themes available for download.
My favorite one is Night Owl+ so let’s install night owl+ theme from extensions.
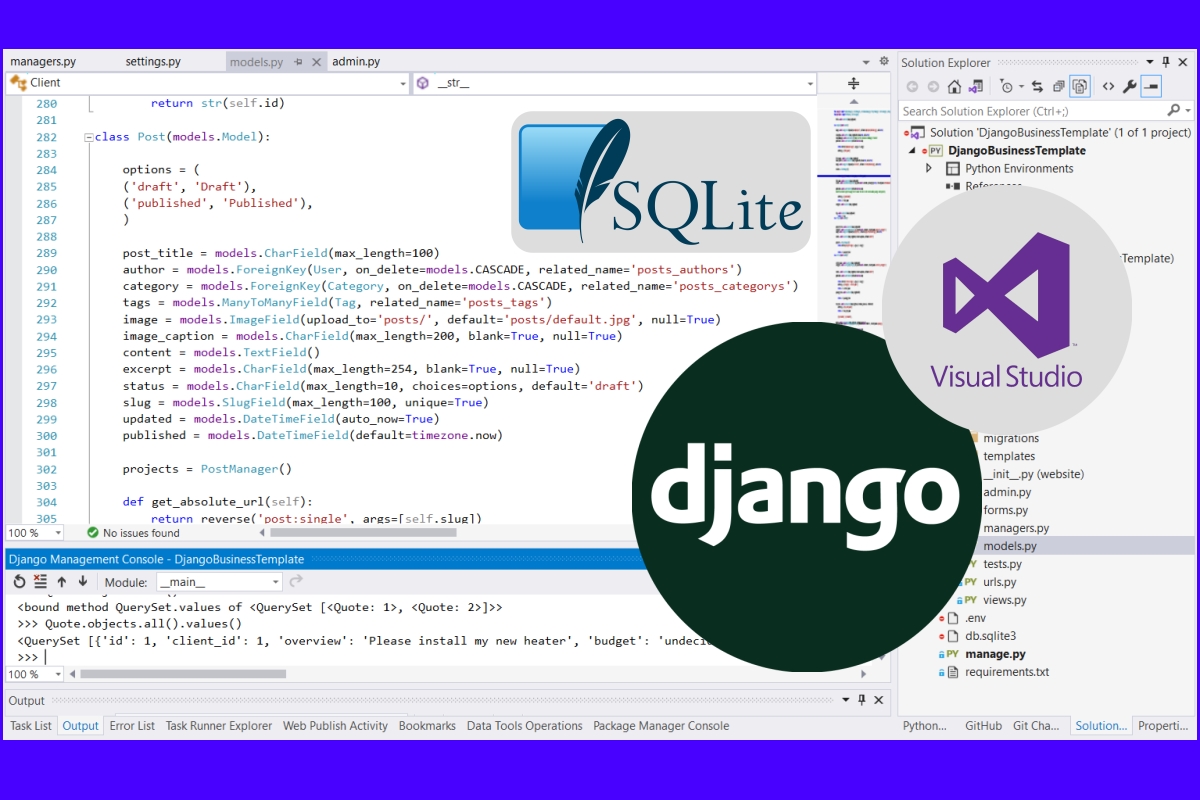
To explore more Vscode themes visit https://vscodethemes.com/
To change the theme go to, file>Preference> color theme
And select the theme that you prefer.
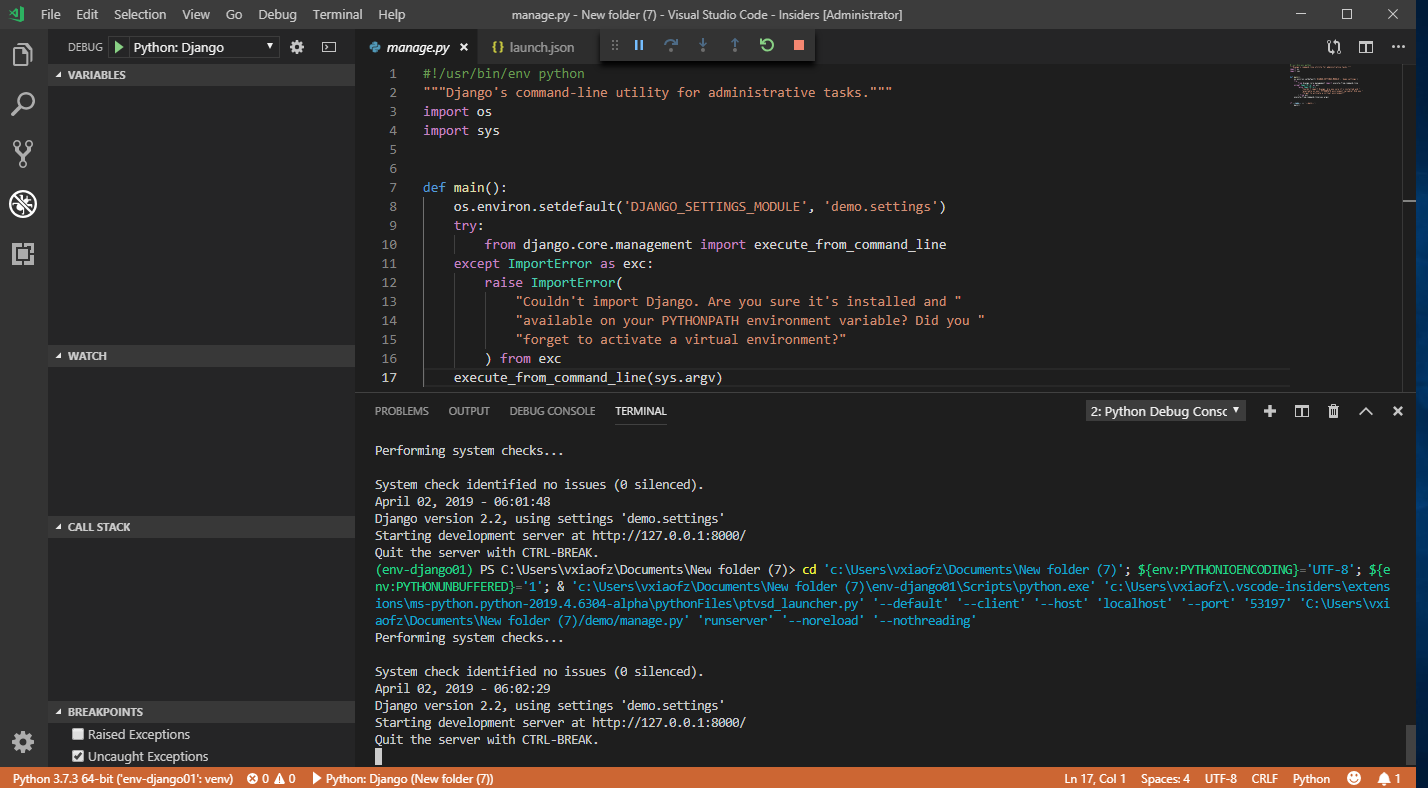
Notice below the color theme there is an option for the file icontheme.
Visual Studio Django Run Manage.py
Download Material Icon Theme from extensions store and click on that and select the icon theme this will give different file icons for different files.

After that restart VS code to activate the extensions. Now open any of your Django project you should get this beautiful view.
Your font might look different I am using Fira code font here.
Configuring Additional Useful Settings
Go to file> preferece> settings then open settings.json from there.
In USER SETTINGS inside the curly braces { }
add the following Settings.
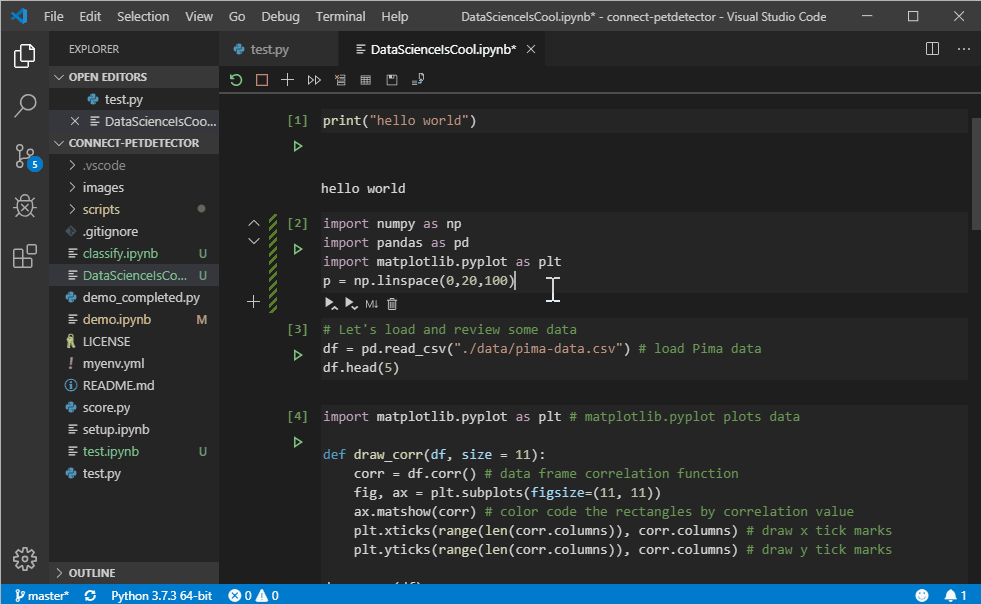
Visual Studio Django
To use the above features, the editor will prompt you to install pylint and autopep8, or you can install them directly in the virtual environment.
Some useful VScode extensions:
Visual Studio Django Unresolved Import
- Code Spell Checker
- Django
- Git History
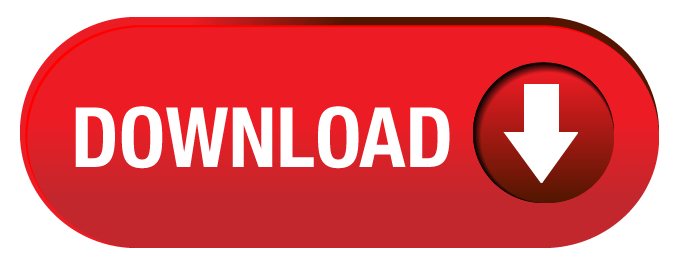